Vue Composition API, Refs, Reactive Syntax, Methods & Functions
Vue refs, reactive syntax, methods and functions are all important as they allow developers to create interactive web applications with ease and maintainability.

- Introduction
- Vue Ref
- Methods & Functions
- Vue Composition API
- Reactive Syntax
- Class Material(s)
- Conclusions
Introduction
Vue Ref, reactive syntax, methods and functions are all essential components of the Vue.js JavaScript framework. They allow developers to create interactive web applications that are highly performant, responsive, and maintainable.
In this blog post, we will explore what Vue ref, reactive syntax, methods and functions are, and provide examples of how to use them.
Vue Ref
Vue refs are a way for developers to access a specific element or component inside the Vue template. Refs are declared using the ref attribute on an element or component and can be accessed within the Vue instance using this.$refs.
For example, let's say we have a Vue component called 'MyComponent'
that contains an element with the ref attribute set to 'myElement'
.
// MyComponent.vue
<template>
<div ref="myElement">
...
</div>
</template>
We can then access the element inside the Vue instance using this.$refs.myElement
Methods and Functions
Methods and functions are used to implement business logic in the Vue instance. Methods are declared inside the Vue instance and can be called from within the template, while functions are declared outside of the Vue instance and can be imported as needed.
For example, let's say we have a simple method called 'sayHello'
that logs 'Hello World!'
to the console when called.
// App.vue
<template>
<div>
<button @click="sayHello">Say Hello</button>
</div>
</template>
<script>
export default {
methods: {
sayHello() {
console.log('Hello World!');
}
}
}
</script>
Here, the sayHello()
method is called when the button is clicked, and the 'Hello World!'
message is logged to the console.
Vue Composition API
The Vue Composition API is a powerful tool for developers to use when building Vue applications. It allows developers to build components that are more powerful, flexible, and easier to maintain. The Composition API was introduced in Vue 3, and provides an alternative to the traditional Options API.
What is Vue Composition API?
The Composition API is an API that allows developers to compose components in a more intuitive and flexible way. It allows developers to write components that are more expressive, more organized, and more maintainable.
The Composition API introduces a set of functions that can be used to create components. These functions allow developers to create components that are more powerful and easier to maintain than those created with the traditional Options API.
How does it work?
The Composition API works by providing a set of functions that can be used to create components. These functions allow developers to create components that are more powerful, flexible, and easier to maintain.
The Composition API functions are split into two categories: setup and composition functions. Setup functions allow developers to define the data, methods, and lifecycle hooks for their components. Composition functions allow developers to combine multiple components together in a single file.
Examples of Vue Composition API
Let’s take a look at a few examples of how to use the Composition API in your Vue applications.
The Setup Function: The setup function allows developers to define the data, methods, and lifecycle hooks for their components.
For example, here’s how you could use the setup function to define a message variable and a greeting method:
// Message.vue
<script>
export default {
setup() {
const message = ref('Hello World!');
function greeting() {
return `${message.value} from Vue Composition API`;
}
return {
message,
greeting
};
}
};
</script>
Composition Function: The composition functions allow developers to combine multiple components together in a single file.
For example, here’s how you could use the composition functions to create a component that contains two other components:
// App.vue
<template>
<div>
<Message />
<User />
</div>
</template>
<script>
import { component } from 'vue';
import Message from './Message.vue';
import User from './User.vue';
export default {
setup() {
const MessageComponent = component(Message);
const UserComponent = component(User);
return {
MessageComponent,
UserComponent
};
}
};
</script>
Reactive Syntax
Reactive syntax is the Vue.js way of expressing data bindings that update the DOM
when the underlying data changes. These data bindings can be expressed in various ways, such as using the v-model directive, the v-bind directive, or by using the ES2015 computed properties.
For example, let's say we have a simple Vue instance that has a data property called 'message'. We can use the v-model directive to create a two-way data binding between the message data property and an input field.
// App.vue
<template>
<div>
<input type="text" v-model="message">
<p>{{ message }}</p>
</div>
</template>
<script>
export default {
data() {
return {
message: 'Hello World!'
}
}
}
</script>
In this example, any changes made to the input field will be automatically reflected in the message data property and vice-versa.
Vue Reactive System
Reactivity is a fundamental concept in Vue.js that allows for automatically updating the UI when data changes. Vue 3's Composition API provides two methods for declaring reactive data: ref and reactive. In this post, we'll explore how to use both methods and when to choose one over the other.
Ref Ref is used to create a reactive variable with a single value. It takes any JavaScript primitive as its initial value, such as a string, number, or boolean. Here's an example:
import { ref } from 'vue'
const count = ref(0)
To access the value of a ref variable, we use the .value
property:
console.log(count.value) // 0
Ref variables can be passed as props or emitted as events in components. Changing the value of a ref variable will trigger a re-render of the component. Here's an example of a button that increments the count
variable when clicked:
<template>
<button @click="increment">{{ count }}</button>
</template>
<script>
import { ref } from 'vue'
export default {
setup() {
const count = ref(0)
const increment = () => {
count.value++
}
return {
count,
increment
}
}
}
</script>
Reactive Reactive is used to create a reactive object with multiple properties. It takes an object as its initial value, where each property can be a reactive variable created with ref
or reactive
. Here's an example:
import { reactive } from 'vue'
const data = reactive({
count: ref(0),
name: 'John Doe'
})
To access a property of a reactive object, we can use dot notation:
console.log(data.count.value) // 0
console.log(data.name) // 'John Doe'
Reactive objects should not be destructured, as the resulting variables will not be reactive. Instead, we should use dot notation to access the properties:
data.count.value++
Here's an example of a button that increments the count
property of a reactive object when clicked:
<template>
<button @click="increment">{{ data.count.value }}</button>
</template>
<script>
import { reactive } from 'vue'
export default {
setup() {
const data = reactive({
count: ref(0)
})
const increment = () => {
data.count.value++
}
return {
data,
increment
}
}
}
</script>
When to use ref vs. reactive
The choice between ref and reactive depends on the specific use case.
- Ref is best suited for creating reactive variables with a single value that can be passed around as props or emitted as events.
- Reactive is best suited for creating reactive objects with multiple properties that are used internally within a component.
In general, it's best to use one consistent pattern for reactive data in a codebase, even if it means sacrificing some convenience.
Class Resource(s)
Conclusion
Vue refs, reactive syntax, methods and functions are all important components of the Vue.js framework. They allow developers to create interactive web applications with ease and maintainability.
Vue 3's Composition API provides two methods for declaring reactive data: ref and reactive.
The choice between ref and reactive depends on the specific use case and personal preference.
Vue Template Refs
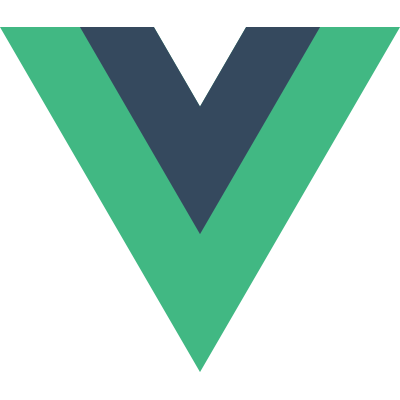
Vue Reactivity Fundamentals
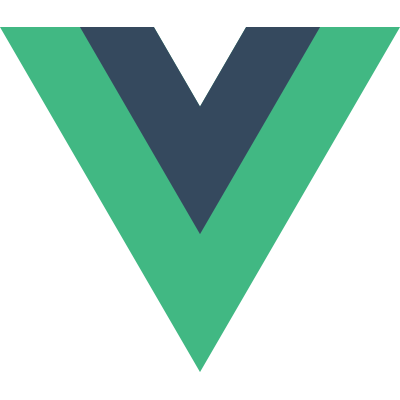
Vue Ref vs Reactive
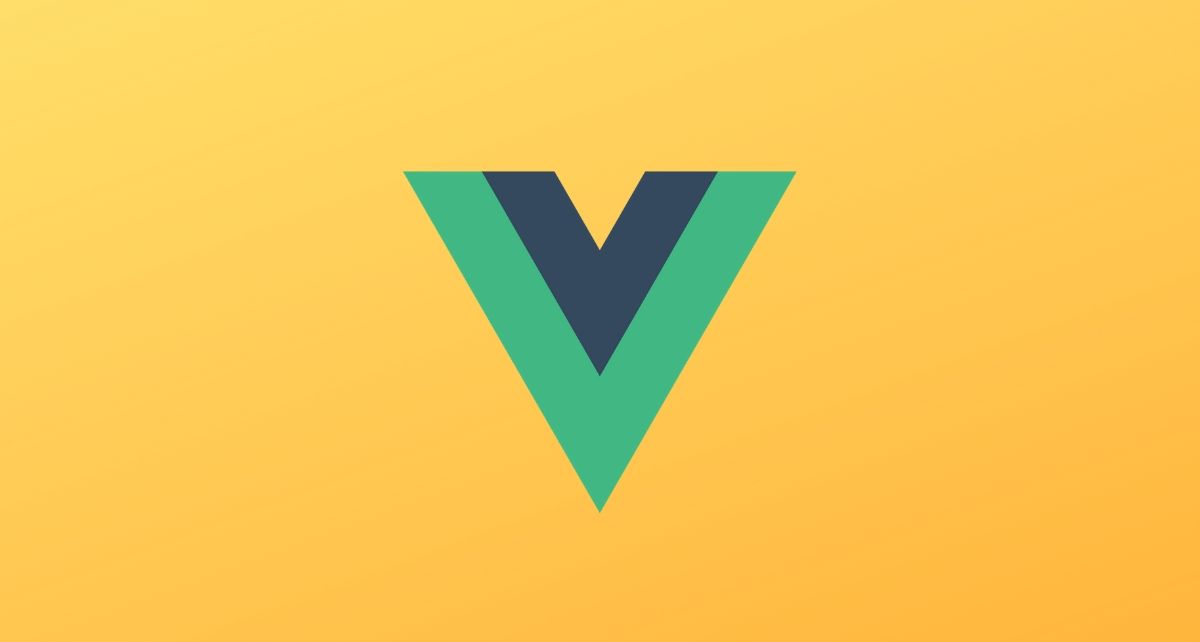