Array Methods: .slice() and .splice()
In JavaScript, slicing and splicing are not about pizza, but they are equally important when it comes to manipulating arrays.
- Introduction
- What is an Array?
- The
slice()
method - Creative Ways to Use slice()
- The splice() Method
- Creative Ways to Use
slice()
- Conclusion
- Useful Resources
Do you love pizza🍕? If you do, then you already understand the concept of slicing and splicing.
Introduction
Imagine you have a large pizza that you want to share with your friends. You can use a pizza cutter to slice the pizza into smaller pieces, that's just how the slice()
method works in JavaScript. It creates a new array that contains a portion of the original array, without modifying the original array.
Now, imagine that you want to add or remove toppings to the pizza. You can use a knife to cut out the unwanted toppings and add new ones, that's same way we use the splice()
method in JavaScript. It can add or remove elements to and from an array, modifying the original array.
Understanding how to use slice()
and splice()
is crucial for working with arrays in JavaScript. With these methods, you can extract specific elements from an array, modify the contents of an array, or even create entirely new arrays.
So, whether you're a pizza lover or not, mastering these methods is an essential skill for any JavaScript developer!👌🏼
What is an Array?
Arrays are a fundamental part of programming in JavaScript, and they come with a set of powerful built-in methods that allow us to manipulate and work with arrays in different ways with ease. Two of these methods are slice()
and splice()
, which are often confused due to their similar names but perform very different operations. In this article, we'll explore the differences between these two methods, their parameters, and common use cases.
Let's GO!
let's dive deeper....

The slice() Method
slice()
is like cutting a slice of pizza! It creates a new array that contains a portion of the original array, without changing the original array. So just like how you can cut a slice of pizza for yourself and still keep the rest of the pizza intact, you can use slice()
to extract specific elements from an array while keeping the original array untouched.
slice()
to take a portion of an array and create a smaller array that contains only the elements you need.The slice()
method returns a shallow copy of a portion of an array into a new array object selected from start
to end
(end
not included) where start
and end
represent the index of items in that array. This method does not change the original array, but rather returns a new array containing a portion of the original array.
Syntax
The syntax for the slice()
method is as follows:
array.slice(start, end)
Explanation
start
- The index at which to begin the slice (inclusive).end
- The index at which to end the slice (exclusive). If omitted, the slice will include all elements from thestart
index to the end of the array.
Example
Let's take a look at an example to see how slice()
works:
const fruits = ['apple', 'banana', 'orange', 'grape', 'kiwi'];
const slicedFruits = fruits.slice(1, 3);
console.log(slicedFruits); // Output: ["banana", "orange"]
console.log(fruits); // Output: ["apple", "banana", "orange", "grape", "kiwi"]const fruits = ['apple', 'banana', 'orange', 'grape', 'kiwi'];
const slicedFruits = fruits.slice(1, 3);
console.log(slicedFruits); // Output: ["banana", "orange"]
console.log(fruits); // Output: ["apple", "banana", "orange", "grape", "kiwi"]
In this example, we have an array of fruits and we're using the slice()
method to create a new array that contains the elements from the fruits
array starting from index 1 (inclusive) to index 3 (exclusive). The slicedFruits
array contains ["banana", "orange"]
, which is a shallow copy of the selected elements from the fruits
array. The original fruits
array remains unchanged.
Creative Ways to Use slice()
There are many creative ways to use slice()
to extract elements from an array. Here are a few common use cases:
- Paginating Data
- Creating Shallow Copies
- Removing Elements
The splice()
Method
splice()
is like adding or removing toppings from a pizza! It can add or remove elements from an array, modifying the original array. Just like how you can add or remove toppings from a pizza to create your own unique combination, you can use splice()
to modify the contents of an array to suit your needs.
splice()
to add or remove elements from an array and create a custom array that fits your needs.The splice()
method is used to add or remove elements from an array, and modifies the original array. Unlike the slice()
method, the splice()
method changes the original array rather than creating a new one.
Syntax
The syntax for the splice()
method is as follows:
array.splice(start, deleteCount, item1, item2, ...)
start
- The index at which to start changing the array. If greater than the length of the array, the actual start index will be set to the length of the array. If negative, it will begin that many elements from the end of the array.deleteCount
- An integer indicating the number of old array elements to remove. If 0, no elements are removed. If greater than the number of elements left in the array starting from start, all the elements through the end of the array will be deleted.item1, item2, ...
- The elements to add to the array, beginning at the start index. If you don't specify any elements, splice() will only remove elements from the array.
Example
Let's take a look at an example to see how splice()
works:
const months = ['January', 'February', 'April', 'June'];
months.splice(2, 0, 'March');
console.log(months); // Output: ["January", "February", "March", "April", "June"]
In this example, we call the splice() method on the months array and pass in the following arguments:
- The index at which to start changing the array (2 in this case)
- The number of elements to remove from the array (in this case, 0, since we're not removing any elements)
- The element(s) to add to the array at the specified index (
March
in this case) - The
splice()
method then adds theMarch
element at index2
, pushing the existing elements to the right.
Creative Ways to Use slice()
There are many creative ways to use slice()
to extract elements from an array. Here are a few common use cases:
- Replacing Element
- Moving Element

Conclusion
In conclusion, slice()
and splice()
are powerful array methods that can help you extract, modify, and move elements in an array.
Whether you're a beginner or an experienced developer, mastering these methods is an essential part of working with arrays in JavaScript.
Useful Resources
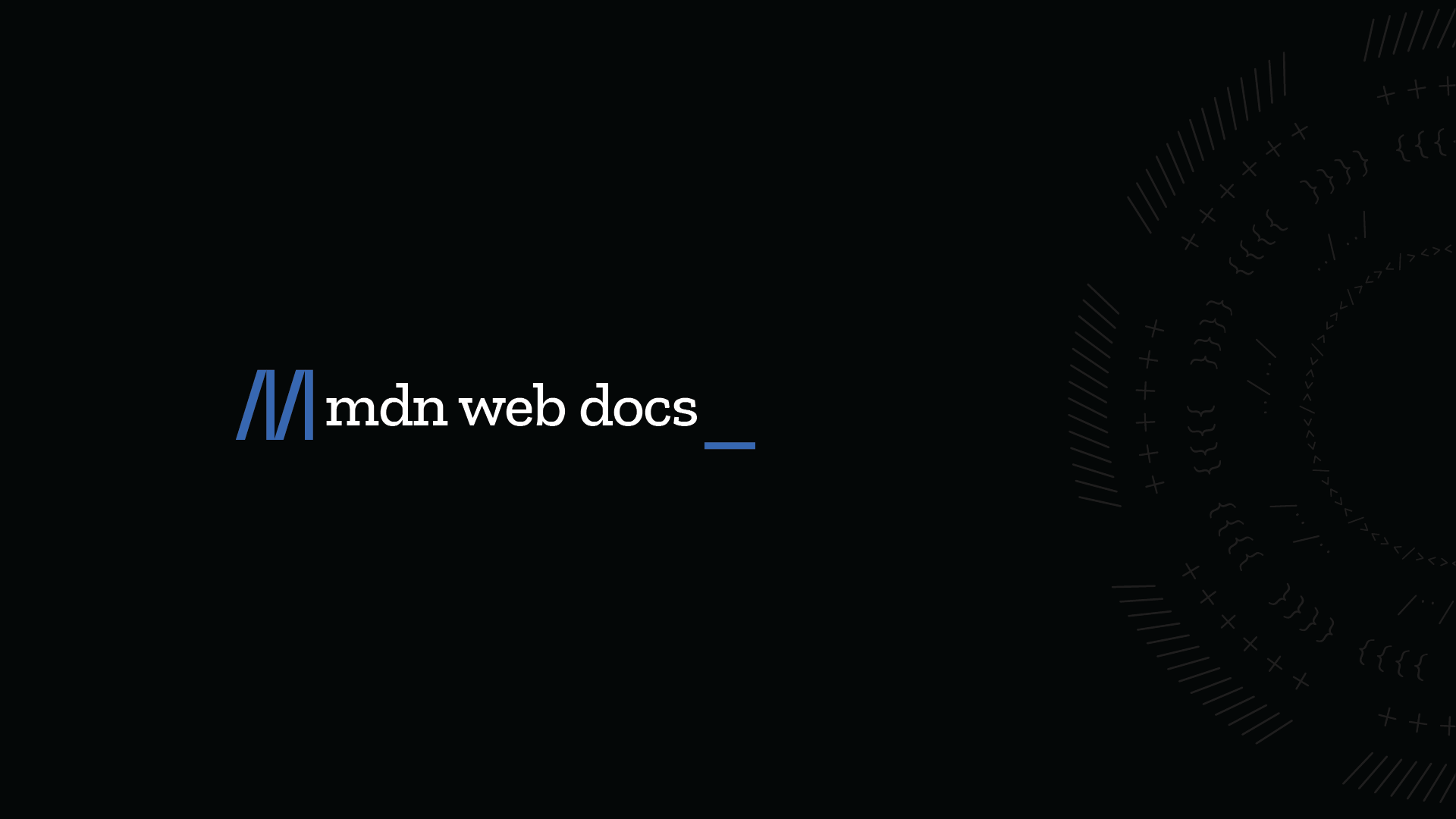
